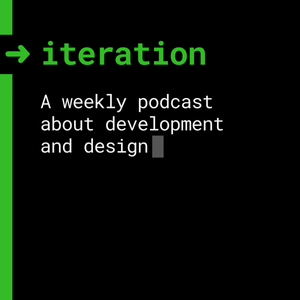
Composition Imposition
02/11/19 • 41 min
Combining Objects with Composition
Metz, Sandi. Practical Object-Oriented Design in Ruby
A weekly podcast about programming, development, and design through the lens of amazing books, chapter-by-chapter.
“Combining the qualities of two existing subclasses, something that inheritance cannot readily accommodate.”
We talked about inheritance, then modules, now this builds on top of the idea of modules and pushes it further.
Composing a bicycle of parts...
Bicycle > has A parts
Parts has subclasses of - RoadBikeParts and MountainBikeParts
Making the Parts Object More Like an Array - This part was freaky
Parts Factory
Different Configs within the parts factory > an array of all the keys and attributes - road_config - mountain_config
Using all this to make a recumbent bike:
Once this is all set up you have this incredibly powerful interface of a bicycle composed of parts:
Bicycle.new(
size: 'L',
parts: PartsFactory.build(recumbent_config))
Composition VS Aggregation
A Meal is composed of an appetizer - An appetizer does not survive outside of the meal. When the meal is gone by definition the appetizer is gone. A meal is composed of an appetizer.
A band is an aggregate of musicians - when the band breaks up the musicians don’t die.
“Composition” encompasses both aggregation and composition -
“This distinction between composition and aggregation may have a little practical effect on your code.”
Deciding Between Inheritance and Composition
“Think of it this way: For the cost of arranging objects in a hierarchy, you get message delegation for free.”
When in doubt use Composition over inheritance
“The general rule is that faced with a problem that composition can solve, you should be biased towards doing so. If you cannot explicitly defend inheritance as a better solution, use composition.”
John’s Pick: Book: "It Doesn’t Have To Be Crazy At Work" -> David Heinemeier Hansen and Jason Fried
JP: Kahn Academy - digging into math again
Next Week: Final Chapter - Designing Cost-Effective Tests
Combining Objects with Composition
Metz, Sandi. Practical Object-Oriented Design in Ruby
A weekly podcast about programming, development, and design through the lens of amazing books, chapter-by-chapter.
“Combining the qualities of two existing subclasses, something that inheritance cannot readily accommodate.”
We talked about inheritance, then modules, now this builds on top of the idea of modules and pushes it further.
Composing a bicycle of parts...
Bicycle > has A parts
Parts has subclasses of - RoadBikeParts and MountainBikeParts
Making the Parts Object More Like an Array - This part was freaky
Parts Factory
Different Configs within the parts factory > an array of all the keys and attributes - road_config - mountain_config
Using all this to make a recumbent bike:
Once this is all set up you have this incredibly powerful interface of a bicycle composed of parts:
Bicycle.new(
size: 'L',
parts: PartsFactory.build(recumbent_config))
Composition VS Aggregation
A Meal is composed of an appetizer - An appetizer does not survive outside of the meal. When the meal is gone by definition the appetizer is gone. A meal is composed of an appetizer.
A band is an aggregate of musicians - when the band breaks up the musicians don’t die.
“Composition” encompasses both aggregation and composition -
“This distinction between composition and aggregation may have a little practical effect on your code.”
Deciding Between Inheritance and Composition
“Think of it this way: For the cost of arranging objects in a hierarchy, you get message delegation for free.”
When in doubt use Composition over inheritance
“The general rule is that faced with a problem that composition can solve, you should be biased towards doing so. If you cannot explicitly defend inheritance as a better solution, use composition.”
John’s Pick: Book: "It Doesn’t Have To Be Crazy At Work" -> David Heinemeier Hansen and Jason Fried
JP: Kahn Academy - digging into math again
Next Week: Final Chapter - Designing Cost-Effective Tests
Previous Episode
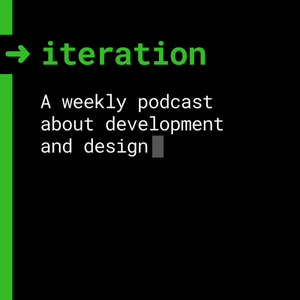
Modules! Modules! Modules!
Metz, Sandi. Practical Object-Oriented Design in Ruby
Chapter 7. Sharing Role Behavior with Modules
Welcome to iteration
A weekly podcast about programming, development, and design through the lens of amazing books, chapter-by-chapter.
Modules! Modules! Modules!
Last episode we talked about inheritance which was kind of an extension of duck typing... but sometimes we need to combine the qualities of two existing subclasses, something that inheritance cannot readily accommodate.
Many object-oriented languages provide a way to define a named group of methods that are independent of class and can be mixed in to any object. In Ruby, these mix-ins are called modules.
Discovering the Schedulable Duck Type
The Schedule expects its target to behave like something that understands lead_days, that is, like something that is “schedulable.” You have discovered a duck type.
This specific example illustrates the general idea that objects should manage themselves; they should contain their own behavior.
Mountain Bike? Mechanic?
Now, in the code above, the dependency on Schedule has been removed from Bicycle and moved into the Schedulable module, isolating it even further.
Like Using Inheritance
COMES BACK TO AUTOMATIC MESSAGE DELEGATION
Loggable Example
When Bicycle includes Schedulable, all of the methods defined in the module become part of Bicycle’s response set.
When a single class includes several different modules, the modules are placed in the method lookup path in reverse order of module inclusion. Thus, the methods of the last included module are encountered first in the lookup path.
When a single class includes several different modules, the modules are placed in the method lookup path in reverse order of module inclusion. Thus, the methods of the last included module are encountered first in the lookup path.
Picks:
John: Type to Siri - Accessibility > Siri > Type to Siri
JP: Scrimba - https://scrimba.com/playlist/pKwrCg
Next Episode
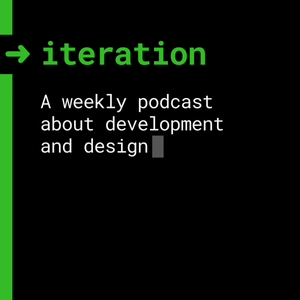
Testing... Testing... 123...
Iteration S05E09
Testing... Testing... 123
Publishing February 18th - Hope everyone had a good Valentine’s Day weekend!
A weekly podcast about programming, development, and design through the lens of amazing books, chapter-by-chapter.
Introduction
- Writing changeable code is an art on which practice relies on three different skills. First, you must understand object-oriented design. Poorly designed code is naturally difficult to change.
- First, you must understand object-oriented design. A poorly designed code is naturally difficult to change.
- Second, you must be skilled at refactoring code.
- Finally, the art of writing a changeable code requires the ability to write high-value tests. Tests give you the confidence to refactor constantly.
Reasons to test:
- Finding Bugs
- Supplying Documentation
- Deferring Design Decisions
- Supporting Abstractions
- Exposing Design Flaws
Knowing What to test:
- “Most developers write too many tests” - OR NONE! hahaha
- Tests should concentrate on the incoming or outgoing messages that cross an object’s boundaries.
- Back to the Kitchen Metaphor - Test that ordering a hamburger, returns a hamburger as expected, not that the kitchen staff turns on the grill or slices tomatoes.
- Incoming messages should be tested for the state they return.
- Outgoing command messages should be tested to ensure they get sent.
- Outgoing query messages should not be tested.
Knowing When to Test
- Write tests first, whenever it makes sense to do so
Knowing How to test:
- Testing Styles: Test Driven Development (TDD) and Behavior Driven Development (BDD).
- Proving the Public Interface
- Injecting Dependencies as Roles
- Testing Inheritance - Test your base class - then include the module to test each of the responses that are in the base class.
- Creating Test Doubles - DiameterDouble - A test double is a stylized instance of a role player that is used exclusively for testing - tend to override the base class in my test helper - I’ve run into silent errors this way.
- Testing Private Methods - interface. These private messages are like proverbial trees falling in empty forests; they do not exist, in a perfect world they do not need to be tested - testing them is a code smell.
- Testing Ducks - create a preferred test interface - mechanic and a guide can both prepare - you can establish a single interface and simply pass the different objects into it.
- Testing Inherited Code
- Testing Models + Objects Vs interface - where’s the balance?
Tests are indispensable. Well-designed applications are highly abstract and under constant pressure to evolve; without tests these applications can neither be understood nor safely changed. The best tests are loosely coupled to the underlying code and test everything once and in the proper place. They add value without increasing costs.
Next Episode - Recap of Practical Object-Oriented Design and New book announcement!
Picks:
NOUN PROJECT - https://thenounproject.com/
**Super Smash Bros Ultimate **
If you like this episode you’ll love
Episode Comments
Generate a badge
Get a badge for your website that links back to this episode
<a href="https://goodpods.com/podcasts/iteration-225636/composition-imposition-25584507"> <img src="https://storage.googleapis.com/goodpods-images-bucket/badges/generic-badge-1.svg" alt="listen to composition imposition on goodpods" style="width: 225px" /> </a>
Copy