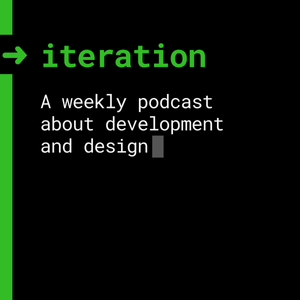
iteration
John Jacob & JP Sio - Web Developers
All episodes
Best episodes
Top 10 iteration Episodes
Goodpods has curated a list of the 10 best iteration episodes, ranked by the number of listens and likes each episode have garnered from our listeners. If you are listening to iteration for the first time, there's no better place to start than with one of these standout episodes. If you are a fan of the show, vote for your favorite iteration episode by adding your comments to the episode page.
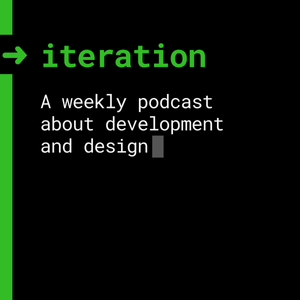
Refactoring Wrap Up & Summary
iteration
07/15/19 • 35 min
Iteration Podcast S06E08
A weekly podcast about programming, development, and design through the lens of amazing books, chapter-by-chapter.
Work Stuff
- Work / Life separation
- Playing a lot with Vue JS - did an implementation on top of rails and loved it.
- Rivets JS
- Pre-built Sketch UI components (https://craftwork.design/)
- Refactoring UI (https://refactoringui.com/)
- Breaking things up! Learning more about technical design patterns.
Summary of "Refactoring"
- The Two Hats - Divide time between adding functionality and refactoring.
- Lots of Nested Functions read like a story
- Refactoring and performance
- Bad Smells
- Catalog of refactors / Catalog of patterns
- Patterns of Enterprise Application Architecture
- Decorator / Presenters (Displays Stuff)
- Query Objects (Looks for stuff)
- Service Objects (Does stuff)
- Gateway Object (Defines access)
- Mapper Object (Pricing Package) that associates customer lease and asset
Picks:
- Send later in Gmail
- Time Timer
- Angeles Crest Highway
Things mentioned
Craftwork design https://craftwork.design/
- Rivests JS
- Vue JS
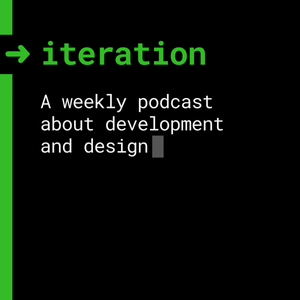
Composition Imposition
iteration
02/11/19 • 41 min
Combining Objects with Composition
Metz, Sandi. Practical Object-Oriented Design in Ruby
A weekly podcast about programming, development, and design through the lens of amazing books, chapter-by-chapter.
“Combining the qualities of two existing subclasses, something that inheritance cannot readily accommodate.”
We talked about inheritance, then modules, now this builds on top of the idea of modules and pushes it further.
Composing a bicycle of parts...
Bicycle > has A parts
Parts has subclasses of - RoadBikeParts and MountainBikeParts
Making the Parts Object More Like an Array - This part was freaky
Parts Factory
Different Configs within the parts factory > an array of all the keys and attributes - road_config - mountain_config
Using all this to make a recumbent bike:
Once this is all set up you have this incredibly powerful interface of a bicycle composed of parts:
Bicycle.new(
size: 'L',
parts: PartsFactory.build(recumbent_config))
Composition VS Aggregation
A Meal is composed of an appetizer - An appetizer does not survive outside of the meal. When the meal is gone by definition the appetizer is gone. A meal is composed of an appetizer.
A band is an aggregate of musicians - when the band breaks up the musicians don’t die.
“Composition” encompasses both aggregation and composition -
“This distinction between composition and aggregation may have a little practical effect on your code.”
Deciding Between Inheritance and Composition
“Think of it this way: For the cost of arranging objects in a hierarchy, you get message delegation for free.”
When in doubt use Composition over inheritance
“The general rule is that faced with a problem that composition can solve, you should be biased towards doing so. If you cannot explicitly defend inheritance as a better solution, use composition.”
John’s Pick: Book: "It Doesn’t Have To Be Crazy At Work" -> David Heinemeier Hansen and Jason Fried
JP: Kahn Academy - digging into math again
Next Week: Final Chapter - Designing Cost-Effective Tests
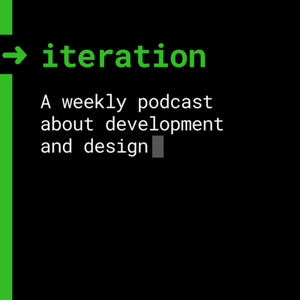
Recap of Pragmatic Programmer
iteration
08/24/18 • 40 min
Pragmatic Programmer in Practice
Welcome to Iteration - A weekly podcast about programming, development, and design through the lens of amazing books, chapter-by-chapter.
In this more casual episode, we recap some of our favorite tips from the Pragmatic Programmer in the context of our recent projects and lessons. We talk though caring about your craft, not leaving any broken windows and more.
Picks:
John - http://www.hemingwayapp.com/
JP - better touch tool
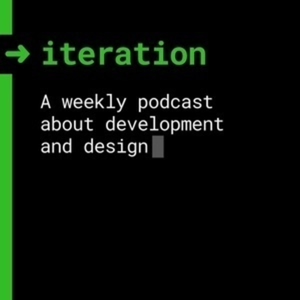
Bend or Break
iteration
06/15/18 • 40 min
Chapter 5 - Bend Or Break
John: Welcome to Iteration: A weekly podcast about programming, development, and design through the lens of amazing books, chapter-by-chapter.
Quick update: Sketch Color Spaces
Today we'll be going through Chapter 5, "Bend or Break" - where we talk about writing flexible code. This is especially important for software engineers in a profession where things are constantly changing. (insert joke about JavaScript frameworks). We will largely focus on different ways we can decouple our code!
Life doesn't stand still. Neither can the code that we write. In order to keep up with today's near-frantic pace of change, we need to make every effort to write code that's as loose—as flexible—as possible.
Part 1 Tip 36: Minimize Coupling Between Modules
Organize code into modules and limit interaction between them. If one module gets compromised and has to be replaced, the other modules should be able to carry on.
Avoid coupling by writing shy code and applying the law of Demeter
What is shy?
- JP: shy doesn't reveal itself to others and doesn't interact with too many people. write shy code. you don't want this: selection.getRecorder().getLocation().getTimeZone()
- John: What does this loo lie practically? Short methods? How do I avoid "coupling?"
Tip 37: Configure, Don't Integrate
Implement technology choices for an application as configuration options, not through integration or engineering
- JP: algo choices, database products, middleware -> these should be configuration options. doing so makes our code more flexible. this is described as "soft" - aka easy to change
- John: Example: Platform fee, or simple basic shared defaults.
Tip 38: Put Abstractions in Code, Details in Metadata
Program for the general case, and put the specifics outside the compiled code base
- JP: think declaratively - not imperatively. specify what, not how. Think about how you write your business logic and business critical things. Think about where you would call these functions or methods. it should be declarative
- John: leave the details as soft—as easy to change—as we can. - "Weekend" automated emails example - valid_send_days
- John: Challenge from book: For your current project, consider how much of the application might be moved out of the program itself to metadata.
Tip 39: Analyze Workflow to Improve Concurrency
Exploit the concurrency in your user's workflow
- JP: temporal coupling - aka coupling in time - aka thinking linear-ly. Decouple your time / order dependencies. ask yourself: what can happen at the same time?. This is a good thought experiment
This example was really good so I'm copying it from the book:
making a pina colada
1 open blender
2 open pina colada mix
3 put mix in blender
4 measure 1/2 cup white rum
5 pour in rum
6 add 2 cups of ice
7 close blender
8 liquefy for 2 mins
9 open blender
10 get glasses
11 get pink umbrellas
12 serve pina coladas
Think about how imperative this is. First do this... then do this...
BUT, think about what you can do concurrently: 1, 2, 4, 10, 11. These can happen all at the same time and up front. Next, 3, 5, and 6 can happen concurrently afterwards. These would be big optimizations
Picks
- JP: Pocket Developer Die
- John: AppSignal - Dropped Rollbar + Scout for this one service.
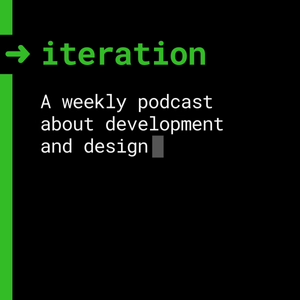
Tracer bullets
iteration
05/11/18 • 29 min
Chapter 2 - A Pragmatic Approach
Overview = combine ideas and processes
- duplicate knowledge throughout systems
- don't split any one piece of knowledge across multiple system components (orthogonality)
- insulate projects from their changing environments
- gather requirements and implement code at the same time
- how to give project estimates
This chapter is truly about a "pragmatic" approach to development
Tip 11: Don't Repeat Yourself
- if you change one, you'll have to change another
Duplicated code arises differently:
- imposed: devs feel they have no choice
- inadvertent: devs don't realize they are duplicating
- impatient: devs got lazy because it seems easier
- interdeveloper: multiple people on a team duplicate a piece of info
we call these the "four i's"
- "shortcuts make for long delays"
- number 4 is a result of large teams - not having a --- dare I say --- a ubiquitous language
- you need good communication to quell number 4
- READ DOCS
- Tip: Lean on code and programmatically generated things to prevent repeating yourself.
Tip 12: Make it easy to reuse
- you want to create an environment where it's easier to find and reuse existing stuff so people don't have to go out and create their own
- orthogonality
- borrowed from geometry
- two lines are orthogonal if they meet at right angles - think axes on a graph
- move along (or parallel to the x-axis) and theres no change to the y-axis
- in computing, "orthogonality" has come to mean independence / decoupling
TWO OR MORE THINGS are orthogonal if changes in one do not affect any of the others
i.e. database code will be orthogonal to the UI. you can change the interface without affecting the database
driving stick shift is not orthogonal
helicopter controls are not orthogonal
bottom line: non-orthogonal systems are hard to maintain
Tip 13: Eliminate Effects Between Unrelated things
- design components that are self-contained. this is very much the case for react components.
- and for ruby methods
- and for js functions
- you want: independent, single, well defined components
- you want: single, independent components that don't need no man
You get two major benefits if you write orthogonal systems: increased productivity and reduced risk.
ways orthogonality can be applied to your work
- teams can be more efficient if each major infrastructure component gets its own subteam: database, communications interface, middleware layer, etc
- non orthogonal systems lead to bickering teams
- system design: should be composed of a set of cooperating modules, each of which implements functionality independent of others
- inherently, i think MVC is orthogonal
- the flux pattern is also orthogonal in react applications. i think? ask yourself:
if i dramatically change the requirements behind a particular function, how many modules are affected?
Toolkits and Libraries
- we're talking Ruby gems, Node modules, etc
- choose technologies wisely
Testing
An orthogonally designed and implemented system is easier to test. Because the interactions between the system's components are formalized and limited, more of the system testing can be performed at the individual module level. This is good news, because module level (or unit) testing is considerably easier to specify and perform than integration testing.
- building unit tests is a TEST of orthogonality.
- easy unit tests == orthogonal
Reversability
Nothing is more dangerous than an idea if it's the only one you have.
Writing more orthogonal code allows you to more easily reverse changess or eb and flow with the needs of the project.
Bottom line: orthognality is about reduction of interdependency among system components
Tip 14: There are no final decisions
- problem: critical decisions aren't easily reversable
- imagine switching a codebase to use Mongo after having tens of thousands of records in a Postgres db
- HOWEVER - if you REALLY abstracted the idea of a "database" out - you should have the flexibility to make that change, should you need to
- you SHOULD prepare for contingencies
instead of carving decisions in stone, think of them mroe as being written in the sand at the beach. A big wave can come along and wipe them out at any time.
- this is also why i love the Adapter pattern.
- for example - making a folder called "Adapters" when making external http requests in a...
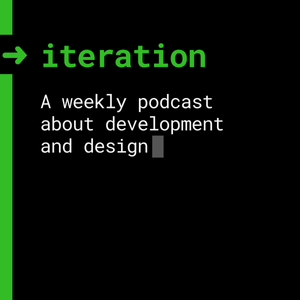
Encapsulation
iteration
06/24/19 • 39 min
Episode 6 - More Code Examples
- Drawing from Chapter 7 - Encapsulation
A weekly podcast about programming, development, and design through the lens of amazing books, chapter-by-chapter.
Encapsulate Record (162)
var organization = { name: "JP Sio", country: "USA" };becomes ⬇️
class Organization { constructor(data) { this._name = data.name; this._country = data.country; } get name() { return this._name; } set name(arg) { this._name = arg; } get country() { return this._country; } set country(arg) { this._country = arg; } }- you can hide what is stored and provide methods
- consumer of class Organization doesn't need to know / care which is stored and which is calculated
- nice getter and setter methods
- makes it easier to refactor -> can hide implementation of internals and update the internals while keeping the same external interface
Encapsulate Collection (170)
class Person { get courses() { return this._courses; } set courses(aList) { this._courses = aList; } }becomes ⬇️
class Person { get courses() { return this._courses.slice(); } addCourse(aCourse) { /*...*/ } }- slice() is key here, does not modify the original array - https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Array/slice
- common approach is to provide a getting method for the collection to return a copy
- basically, never mutate the original
Replace Primative with Object (174)
orders.filter(0 => "high" === o.priority || "rush" === o.priority)becomes ⬇️
orders.filter(o => o.priority.higherThan(new Priority("normal")));- this goes back to "Primitive Obsession"
- programmers are often hesitant to create their own types and rely only on primitives. i.e. representing a phone number as a string instead of as it's own type
A telephone number may be represented as a string for a while, but later it will need special behavior for formatting, extracting the area code, and the like
- create a new class for that bit of data
- at first, the class does very little. in fact it probably only wraps a primitive
- but now you have a place to put behavior specific to its needs
Inline Function (115) 🥴
Sometimes it's better to not try to split things apart, sometimes it just complicates things.
// before refactor: function getItemPrice(item) { if (itemOnSale(item) == true) { return item.price - 5 } else { return item.price } }; function itemOnSale(product) { if (product.onSale == true) { return true; } else { return false; } }; let original = getItemPrice(sweatshirt); // after refactor: function newGetItemPrice(item) { if (item.onSale == true) { return item.price - 5 } else { return item.price } };Extract Class (182) 🥴
- Talk through HUGE applicant model (in Ruby)
- Broke this into child objects
- Applicant Health History
- Applicant Habits
- Applicant Lifestyle
- Applicant Method
- Applicant Legal Release
Picks
- JP: None :(
- John: Quad Lock phone mount - bikes
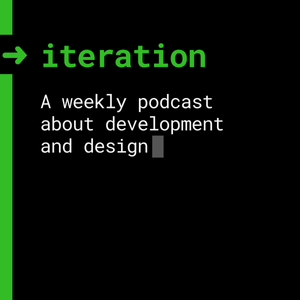
💬 Technical Interviews
iteration
04/27/21 • 49 min
Welcome to Iteration, a podcast about programming, development, and design
John has been asked:
- When I perform a google search, what happens? Be as specific and accurate as possible including every layer of technology.
- Can you tell me what Indexes are and what they do?
- What is CORS?
Questions JP has been asked:
- What is the difference between something like SQL and Mongo - what are the trade offs?
- How does the JS bridge work in React Native?
- Describe what Redux is for and how you'd implement it in a React project
Maybe some from our own?
- If you could add one feature or change to the Rails framework what would it be?
- How do feel about testing? How do you think about testing? When does it make sense to write tests?
- If I have a really huge model, let's say 500+ lines, how would you go about refactoring it? Example link: https://github.com/discourse/discourse/blob/master/app/models/post.rb
Picks
- I used this to pair with Joe and it was SICK
John: YNAB (https://www.youneedabudget.com/)
- Different approach to budgeting that sucks less
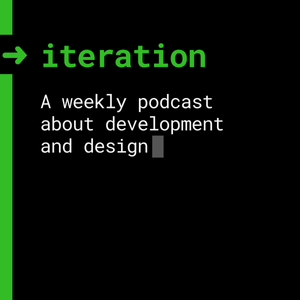
Does Tech Stack Matter? 🥞
iteration
12/07/20 • 39 min
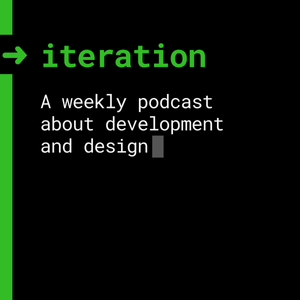
The Soft Skills Episode 🍦
iteration
06/15/20 • 37 min
Welcome to Iteration, a weekly podcast about programming, development, and design.
- JP Intro — Hi, I'm JP and I am a full stack developer. Today, I am joined by John:
- John Intro — My name is John and I am a software developer for a home services startup.
What are soft skills? Why are they important? Are they important?
Wikipedia defines "soft skills" ...
Soft skills are a combination of people skills, social skills, communication skills, character or personality traits, attitudes, career attributes, social intelligence and emotional intelligence quotients, among others, that enable people to navigate their environment, work well with others, perform well, and achieve their goals with complementing hard skills.
tldr; people skills
Hard skills, also called technical skills, are any skills relating to a specific task or situation. It involves both understanding and proficiency in such specific activity that involves methods, processes, procedures, or techniques
Conversation is loosely based on this book, the author is famously kind of a dick. Doesn't mean there aren't some solid takeaways, using it as a framework for conversation.
- Link to summary of "Soft Skills"
- Another summary
Section 1: Career
Few tips to improve your career:
- From SS: Specialize, don't generalize.
- From SS: "Fake it till you make it"
John
- Always be working on yourself: "Luck is when preparation meets opportunity"
- Meet lots of people, be helpful and friendly
- Do a lot of interviews.
- Confidence and enthusiasm — "Being enthusiastic is worth 25 IQ points."
JP
- The importance of friendliness. How does this work for introverts?
Recommended Career Books
Section 2: Marketing yourself
- From SS: See yourself more as offering a service and not as a employee.
- Less about Salary and work hours, more about the uniqe "Features and beniftis" you bring to the table, you solve problems, you are an investment not an expense.
John
- Blog, be vocal — Share what you learn, don't be afraid to look dumb.
- Teach others when you can
- Take speaking and presentation gigs (Was a speaker at GA and got work out of it, you never know)
- Again — Specialize
JP
- I love this idea around you being a service. EAAS: Engineer as a service
- I have mixed feelings about marketing yourself. I go back and forth on whether or not I want a bigger online presence
Section 3: Learning
From SS: "Learn you want? Teach you must."
John:
- Be consistent. 1 hour a day for 12 days is way better than a single 12 hour day.
- Try to understand the concepts, not the syntax.
- Concepts and fundamentals you can take anywhere. Good domain design, testing, clean code. All these concepts work in any language / framework.
JP:
- Deliberate practice. I just hammer concepts into my brain until it sticks.
- Honestly, just keep writing code but more importantly keep READING code
- Whiteboard, talk about problems from a domain perspective
Section 4: Productivity
From SS: Focus
- John: +1 (20% done isn't worth anything. 5 tasks 20% done, or 1, 100% done)
From SS: Pomodoro Technique
From SS: Kanban
John:
- Getting Things Done
- Break down the work.
- 80/20 — Pareto principle
- Eat that Frog
JP:
- I'm currently giving Pomodoro a shot. I'm trying to figure out how to effectively context shift
- How do you eat an elephant?
Sections 5 and 6 — Financial and Fitness
Section 7: Spirit
From SS: power of positive thinking
From John:
- Mental space, day off, unplug sometimes.
- Confidence + enthusiasm
JP:
- I could use some tips from this section...
BONUS From John: Commu...
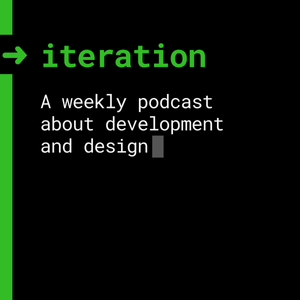
08/12/19 • 50 min
Season 7 Epsiode 2
A weekly podcast about programming, development, and design through the lens of amazing books, chapter-by-chapter
5 Essential Values in Extreme Programing
Extreme Programing By Kent Beck - Chapters 2,3 and 4
Chapter 2 - Learning to Drive
frequent, small corrections
don't wait to find out if you are going in the wrong direction
Chapter 3 - Values, Principles, and Practices
values are the roots of things we like and don't like in a situation.
Making values explicit is important because without values, practices quickly become rote (habitual repetition), activities performed for their own sake buck lacking any purpose or direction.
practices are evidence of values
Practices are clear. Everyone knows if I've attended the morning standup meetings. Whether I really valuecommunication is fuzzy. Whether I maintain practices that enhance communication is concrete.
principles bridge the gap between values and practices
START HERE
Chapter 4 - Values
Chapters 2 and 3 are small introductory sections, here is the TLDR:
Software, teams, and requirements change. We need to be able to adapt
to such change. The next 3 sections will be about values, practices,
and principles of Extreme Programming
Chapter 4 is about values
Everyone who touches software has a sense of what matters. One person might think what really matters is carefully thinking through all conceivable design decisions before implementing. Another might think what really matters is not having any restrictions on his own personal freedom.
What actually matters is not how any given person behaves as much as how the individuals behave as part of a team and as part of an organization.
Sometimes it's easy to bikeshed over things like code style. Arrow functions vs function declaration, inline functions vs methods, etc
XP embraces 5 values to guide development
- Communication
- Simplicity
- Feedback
- Courage
- Respect
- Communication
When you encounter a problem, ask yourselves if the problem was caused by a lack of communication. What communication do you need now to address the problem? What communication do you need to keep yourself out of this trouble in the future?
JP: Retro feedback and postmortem feedback often includes communication - both good and bad
2. Simplicity
To make a system simple enough to gracefully solve only today's problems is hard work.
"What is the simplest thing that could possible work?"
JP: Emphasis on "today's problems" - how can we provide value to the customer without compromising on theirexperience and without overengineering a solution?
JS: Stakeholder communicatinon, actively work to include everyone in at the key touchpoints, initial concept, mokcup, prototype, final review.
JS: Have something to look at / talk about. Somebody make a mockup, somebody write some pseudocode
- Feedback
Being satisfied with improvement rather than expecting instant perfection, we use feedback to get closer and closer to our goals. Feedback comes in many forms.
opinions about an idea, yours or your teammates
how the code looks when you implement the idea
whether the tests were easy to write
whether the tests run
how the idea works once it has been deployed
JP: Be agile! Look to build an MVP. Get analytics on things and make decisions from there. It's hard to invest in an idea entirely and then end up ditching the whole thing. Everyone feels burned.
JS: Again, think through key touchpoints, (99%, 50%, 1%)[https://medium.com/the-mission/how-to-scale-yourself-the-99-50-1-framework-7798518f36e1]
- Courage
Courage as a primary value without counterbalancing values is dangerous. Doing something without regard for the consequences is not effective teamwork. [...] The courage to speak truths, pleasant or unpleasant, fosters communication and trust. The courage to discard failing solutions and seek new ones encourages simplicity. The courage to seak real, concrete answers creates feedback.
JP: Being able to speak up when you disagree with people's ideas and being able to listen and exercising patience.
JS: Being honest with yourslef and stakeholders about timelines, replytimes, scope.
JS: Having the courage to set boundries. - "I need this by tomorrow" - I'm sorry, I can't make that happen for you.
5. Respect
If members of a team don't care about each other and what they're doing, XP won't work. If members of a team don't care about a project, nothing can save it. Every person whose life is touched by software development has equal value as a human being. No one is intrinsically worth more than anyone else.
JP: It's much easier when everyone respects each other.
JS: Reconize there's rarely a "wrong"...
Show more best episodes

Show more best episodes
FAQ
How many episodes does iteration have?
iteration currently has 78 episodes available.
What topics does iteration cover?
The podcast is about Code, Javascript, Design, Web Development, Ruby, Software Development, Podcasts, Technology and Business.
What is the most popular episode on iteration?
The episode title 'Engineering Management 🔨' is the most popular.
What is the average episode length on iteration?
The average episode length on iteration is 44 minutes.
How often are episodes of iteration released?
Episodes of iteration are typically released every 7 days.
When was the first episode of iteration?
The first episode of iteration was released on May 4, 2018.
Show more FAQ

Show more FAQ