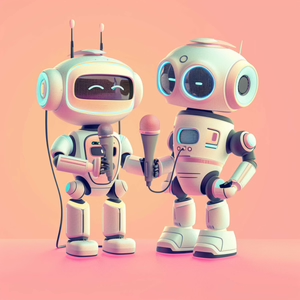
Java Internals Crashcasts
Fatih Yavuz
All episodes
Best episodes
Top 10 Java Internals Crashcasts Episodes
Goodpods has curated a list of the 10 best Java Internals Crashcasts episodes, ranked by the number of listens and likes each episode have garnered from our listeners. If you are listening to Java Internals Crashcasts for the first time, there's no better place to start than with one of these standout episodes. If you are a fan of the show, vote for your favorite Java Internals Crashcasts episode by adding your comments to the episode page.
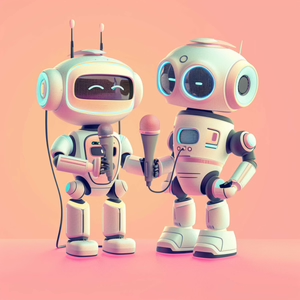
Mastering the Exchanger: Key to Efficient Systems
Java Internals Crashcasts
09/07/24 • 7 min
Dive into the world of Java concurrency with an in-depth exploration of the Exchanger class, a powerful tool for synchronizing thread interactions.
In this episode, we explore:
- The fundamentals of Exchanger and its role in concurrent programming
- Real-world analogies and use cases for Exchanger in pipeline designs
- Potential pitfalls and limitations, including handling odd thread numbers
- Best practices for effective Exchanger implementation in your projects
Tune in to master this key synchronization utility and elevate your Java concurrent programming skills!
Want to dive deeper into this topic? Check out our blog post here: Read more
★ Support this podcast on Patreon ★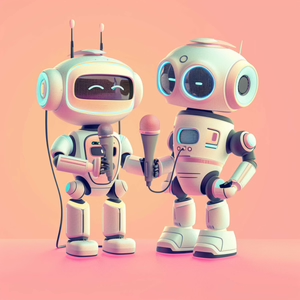
Harnessing the Power of CompletableFuture: Mastering Asynchronous Java Programming
Java Internals Crashcasts
09/07/24 • 7 min
Dive into the world of asynchronous Java programming with CompletableFuture, a powerful tool for managing complex concurrent operations.
In this episode, we explore:
- The advantages of CompletableFuture over regular Futures
- Creating and chaining asynchronous operations with elegance
- Robust error handling techniques for bulletproof code
- Advanced features like combining multiple CompletableFutures
Tune in to unlock the full potential of CompletableFuture and revolutionize your approach to asynchronous programming in Java.
Want to dive deeper into this topic? Check out our blog post here: Read more
★ Support this podcast on Patreon ★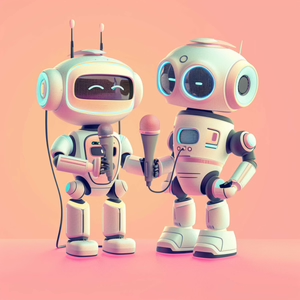
Java's ScheduledExecutorService: Mastering Timed Tasks in Your Code
Java Internals Crashcasts
09/07/24 • 8 min
Dive into the world of timed task management in Java with ScheduledExecutorService, a powerful tool for scheduling and executing tasks with precision.
In this episode, we explore:
- ScheduledExecutorService: Its purpose and common use cases in Java applications
- Key scheduling methods: Understanding the differences between fixed rate and fixed delay
- Best practices and potential pitfalls when working with scheduled tasks
- How ScheduledExecutorService compares to the older Timer and TimerTask classes
Tune in to master the art of efficient task scheduling and elevate your Java concurrency skills.
Want to dive deeper into this topic? Check out our blog post here: Read more
★ Support this podcast on Patreon ★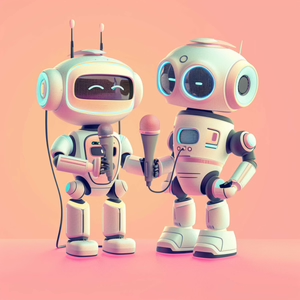
Understanding the Difference: Java's Stack vs. Heap Memory
Java Internals Crashcasts
08/31/24 • 5 min
Dive into the intricacies of Java memory management with our expert guest, Victor, as we unravel the differences between stack and heap memory.
In this episode, we explore:
- The fundamental distinctions between stack and heap memory allocation
- Lifecycle management: How stack frames and heap objects are created and removed
- Performance implications and optimization techniques for efficient memory usage
- Common pitfalls and misconceptions that trip up developers in interviews
Tune in for an in-depth discussion on these crucial concepts and gain valuable insights for your next Java backend engineer interview!
Want to dive deeper into this topic? Check out our blog post here: Read more
★ Support this podcast on Patreon ★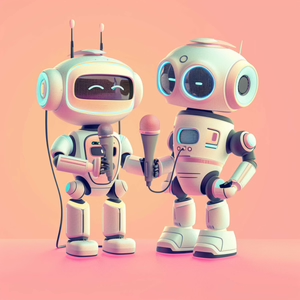
ConcurrentLinkedDeque: Exploring Java's Thread-Safe Double-Ended Queue
Java Internals Crashcasts
09/07/24 • 7 min
Dive into the world of concurrent collections with an in-depth exploration of Java's ConcurrentLinkedDeque.
In this episode, we explore:
- The power of thread-safe, non-blocking double-ended queues
- ConcurrentLinkedDeque vs. LinkedList and ConcurrentLinkedQueue
- Performance considerations and common pitfalls to avoid
- Best practices for leveraging ConcurrentLinkedDeque in your code
Tune in for expert insights, practical tips, and even a fascinating historical tidbit about this powerful data structure!
Want to dive deeper into this topic? Check out our blog post here: Read more
★ Support this podcast on Patreon ★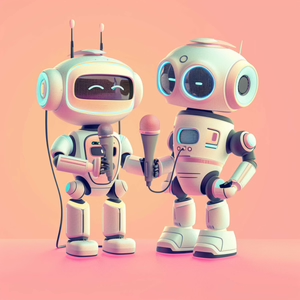
Mastering CopyOnWriteArraySet: Thread-Safe Collections for Concurrent Java Programming
Java Internals Crashcasts
09/07/24 • 7 min
Dive into the world of thread-safe collections with our in-depth exploration of CopyOnWriteArraySet in Java's concurrent programming landscape.
In this episode, we explore:
- The inner workings of CopyOnWriteArraySet and its copy-on-write semantics
- Ideal use cases and performance considerations in concurrent environments
- Common pitfalls and best practices for effective implementation
- How CopyOnWriteArraySet compares to other Set implementations
Tune in for expert insights, real-world examples, and a quiz to test your understanding of thread-safe collections!
Want to dive deeper into this topic? Check out our blog post here: Read more
★ Support this podcast on Patreon ★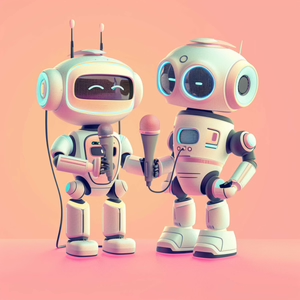
CopyOnWriteArrayList: Java's Thread-Safe Solution for Concurrent Collections
Java Internals Crashcasts
09/07/24 • 8 min
Dive into the world of concurrent collections with an in-depth exploration of Java's CopyOnWriteArrayList.
In this episode, we explore:
- The inner workings of CopyOnWriteArrayList and its thread-safe design
- Performance trade-offs: lightning-fast reads vs. costly writes
- Real-world applications in event-driven programming and caching
- Key differences from other List implementations and potential pitfalls
Tune in to uncover the intricacies of this powerful data structure and learn how to leverage its unique properties in your concurrent programming projects.
Want to dive deeper into this topic? Check out our blog post here: Read more
★ Support this podcast on Patreon ★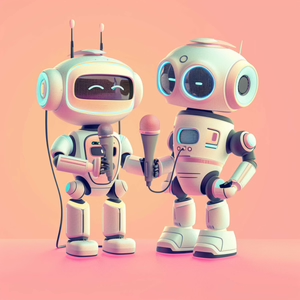
Mastering ConcurrentMap: Thread-Safe, High-Performance Java Collections
Java Internals Crashcasts
09/07/24 • 8 min
Dive into the world of thread-safe Java collections with an in-depth exploration of the ConcurrentMap interface.
In this episode, we explore:
- The fundamentals of ConcurrentMap and its role in concurrent programming
- Atomic operations and essential methods for efficient multi-threaded data manipulation
- Thread-safety mechanisms and real-world applications of ConcurrentMap
Tune in for expert insights on mastering concurrent collections in Java, common pitfalls to avoid, and tips for optimizing your multi-threaded applications.
Want to dive deeper into this topic? Check out our blog post here: Read more
★ Support this podcast on Patreon ★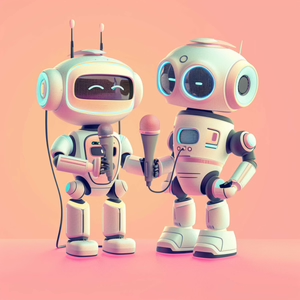
Understanding ArrayBlockingQueue: Java's Thread-Safe Bounded Collection
Java Internals Crashcasts
09/07/24 • 6 min
Dive into the world of Java's concurrent collections with an in-depth exploration of ArrayBlockingQueue, a powerful thread-safe bounded queue implementation.
In this episode, we explore:
- The key characteristics of ArrayBlockingQueue, including its bounded nature and FIFO behavior
- How to create and configure ArrayBlockingQueue, including options for fairness policies
- The difference between blocking and non-blocking operations, and when to use each
- How ArrayBlockingQueue compares to other BlockingQueue implementations like LinkedBlockingQueue
Tune in to uncover practical use cases and gain valuable insights into managing concurrent operations with ArrayBlockingQueue!
Want to dive deeper into this topic? Check out our blog post here: Read more
★ Support this podcast on Patreon ★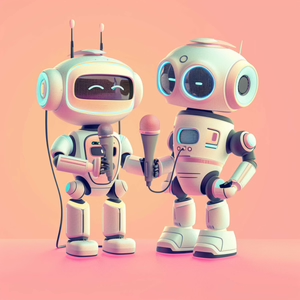
Java's ConcurrentSkipListMap: Mastering Thread-Safe Sorting in Concurrent Applications
Java Internals Crashcasts
09/07/24 • 7 min
Dive into the world of thread-safe sorting with Java's ConcurrentSkipListMap in this illuminating episode of Java Internals Crashcasts!
In this episode, we explore:
- The ingenious skip list structure powering ConcurrentSkipListMap
- How it achieves thread-safety using a lock-free algorithm
- Real-world applications, from priority queues to financial order books
- Common pitfalls and best practices for optimal usage
Join hosts Sheila and Victor as they unravel the complexities of this powerful concurrent collection, revealing its O(log n) efficiency and unique features.
Want to dive deeper into this topic? Check out our blog post here: Read more
★ Support this podcast on Patreon ★Show more best episodes

Show more best episodes
FAQ
How many episodes does Java Internals Crashcasts have?
Java Internals Crashcasts currently has 77 episodes available.
What topics does Java Internals Crashcasts cover?
The podcast is about Learning, Podcasts, Technology, Education and Java.
What is the most popular episode on Java Internals Crashcasts?
The episode title 'Deep Dive into VarHandle and Enhanced Atomics: Boosting Java Concurrency' is the most popular.
What is the average episode length on Java Internals Crashcasts?
The average episode length on Java Internals Crashcasts is 7 minutes.
When was the first episode of Java Internals Crashcasts?
The first episode of Java Internals Crashcasts was released on Aug 31, 2024.
Show more FAQ

Show more FAQ